Deva's Blog
OOP344 Wiki: tvirutthasalam
IRC: gobihun
Saturday, February 11, 2012
MOVED TO WORDPRESS....
Thursday, February 9, 2012
STRING ARRAY CLASS
STRING.CPP
/***************************************************/ /* File : string.h */ /* Writen by: Thevakaran Virutthasalam */ /***************************************************/ #ifndef _TV_STRING_H_ #define _TV_STRING_H_ class StrArr{ char* _string; unsigned int _size; void AloCopy(const StrArr&); public: StrArr (unsigned int); StrArr (const StrArr&); StrArr& operator=(const StrArr&); StrArr& operator=(const char*); StrArr& operator=(const char); StrArr& operator+=(const StrArr&); StrArr& operator+=(const char*); StrArr& operator+=(const char); friend StrArr operator+(const StrArr&, const StrArr&); friend StrArr operator+(const StrArr&, const char*); friend StrArr operator+(const StrArr&, const char); virtual ~StrArr(); char& StrArr::operator[](unsigned int); unsigned int size()const; }; char* Strcat(char*, const char*); char* Strcpy(char*, const char*); int Strlen(const char*); int Strcmp(const char*,const char*); #endif
/***************************************************/ /* File : string.cpp */ /* Writen by: Thevakaran Virutthasalam */ /***************************************************/ #include "string.h" void StrArr::AloCopy(const StrArr& X){ _string = new char[X.size()+1]; for(unsigned int i=0;i
_size = X.size(); } StrArr::StrArr(unsigned int size){ //Initialize the object with given size _string = new char[size+1]; *_string = '\0'; _size = size; } StrArr::StrArr(const StrArr& X){ //Initialize the object with given of another object AloCopy(X); } StrArr& StrArr::operator=(const StrArr& str){ //Assigning operator for string object if(this != &str){ delete[] _string; AloCopy(str); } return *this; } StrArr& StrArr::operator=(const char* s ){ //Assigning operator for null terminated string unsigned int size = Strlen(s); if(_size (size*3)){ char* new_string = new char[size*2+1]; delete[] _string; _string = new_string; _size = size*2; } Strcpy(_string, s); return *this; } StrArr& StrArr::operator=(const char c){ //Assigning operator for a single char if(_size < 2 || _size > 100) { //Checking to add/remove enough memory. delete [] _string; char* new_char = new char[11]; _size = 10; _string = new_char; } *_string= c; *(_string+1)='\0'; return *this; } StrArr& StrArr::operator+=(const StrArr& X){ //Appending operator for string object unsigned int i; unsigned int j; char* new_string = new char[X.size()+_size+1]; for(i=0;_string[i]!='\0' && i<_size;new_string[i]=_string[i++]); for(j=0;X._string[j]!='\0' && j<=X.size();new_string[j+i]=X._string[j++]); new_string[j+i]='\0'; delete[] _string; _size += X.size(); _string = new_string; return *this; } StrArr& StrArr::operator+=(const char* s){ //Appending operator for null terminated string unsigned int i; unsigned int j; unsigned int len=Strlen(s); char* new_string = new char[len+_size+1]; for(i=0;_string[i]!='\0' && i<_size;new_string[i] = _string[i++]); for(j=0;s[j]!='\0';new_string[j+i] = s[j++]); new_string[j+i]='\0'; delete[] _string; _size += len; _string = new_string; return *this; } StrArr& StrArr::operator+=(const char c){ //Appending operator for a sigle char unsigned int i = Strlen(_string); if (i+1<_size) { char* new_string = new char[_size+2]; for(i=0;_string[i]!='\0' && i<_size;new_string[i] = _string[i++]); new_string[i++]=c; new_string[i]='\0'; delete[] _string; _size += 1; _string = new_string; } else { _string[i]=c; _string[i+1]='\0'; } return *this; } StrArr operator+(const StrArr& lhs, const StrArr& rhs){ StrArr copyArr = lhs; copyArr += rhs; //using append operator of object return copyArr; } StrArr operator+(const StrArr& lhs, const char* s){ StrArr copyArr = lhs; copyArr += s; //using append operator of string return copyArr; } StrArr operator+(const StrArr& lhs, char c){ StrArr copyArr = lhs; copyArr += c; //using append operator of char return copyArr; } char& StrArr::operator[](unsigned int index){ //Index operator to ruturn char at given index position //of the string if(index >= _size){ char* new_string = new char[index*2 + 1]; for(unsigned int i=0;i<_size;new_string[i] = _string[i++]); delete[] _string; _size = index*2; _string = new_string; } return _string[index]; } unsigned int StrArr::size()const{ //return the size of current object. return _size; } int Strlen(const char* str){ //String length function int i; for(i=0; str[i] != '\0'; i++); return i; } char* Strcpy(char* dest, const char* source){ //String copy function int i; for(i=0;source[i]!='\0';i++){ dest[i] = source[i]; } dest[i]='\0'; return dest; } char* Strcat(char* dest, const char* srce){ unsigned int i = Strlen(dest); Strcpy(dest+i, srce); return dest; } int Strcmp(const char* str1, const char* str2){ //String compare function int result=0; for(int i=0; !result && str1[i] != '\0' && str2[i] != '\0'; i++){ if(str1[i] > str2[i]){ result = 1; } else if(str1[i] < str2[i]){ result = -1; } } return result; } StrArr::~StrArr(){ //Distructor of StrArr object delete[] _string; }
Wednesday, February 1, 2012
Wednesday, August 5, 2009
How to Jailbreak iPhone 3.0.1
The iPhone Dev team released their much awaited software solution earlier to Jailbreak iPhone 3GS OS 3.0 with RedSn0w. However Apple has released 3.0.1 which included the iPhone SMS vulnerability patch recently. So here is a step-by-step guide to jailbreak iPhone 3G(S) using RedSn0w 0.8 (on Windows). For unlocking your iPhone 3G/3GS first jailbreak with following guide, after a successful jailbreak follow our guide on Unlocking iPhone 3GS with UltraSn0w.
After unlocking with Ultrasn0w on 3GS reset your network setting once from Settings->General->Reset->Reset Network settings once. The process is very similar as iPhone 2G, 3G and 3GS jailbreaking.
Download of Interest :
iTunes 8.2: Download here
RedSn0w 0.8: Download here
iPhone 3G Firmware 3.0:Download here
iPhone 3GS Firmware 3.0:Download here
Once you have completes all download then read this guide twice or thrice before doing anything.
Step One : Create a folder on desktop and name it whatever you wants in the following article we named it iPawnage. Now put all your downloaded files in the iPawnage folder. Now connect your iPhone to the computer and launch RedSn0w from iPawnage folder. In the Window click on the browse button to navigate to the iPawnage folder on your desktop and select the 3.0 firmware ipsw.
Step Two : Click the Open button to continue
Step Three : In next Window check the Install Cydia option (remove check on Install Icy) to Jailbreak your iPhone 3G(S).
Step Four :
For iPhone 3G turn off your iPhone but make sure it is still plugged in to USB port. If you satisfies this term then click next to put your iPhone in to DFU mode follow the onscreen instructions as follows Hold the Power button for 3 seconds, Hold the Home and Power buttons for 10 seconds, then release the Power button and continuing holding the Home button until your iPhone is in DFU mode.
For iPhone 3GS Disconnect your iPhone from your computer and turn off it by holding the Power button until 'Slide to Power Off' appears, and the slide to power off. Wait five seconds after the device is fully powered down. While holding down the the Home button, plug the USB cable into the device. KEEP holding down Home until the Apple logo disappears.
Step Five : If all goes well means you put your iPhone in DFU mode successfully then the following screen will appear otherwise you will be prompted to do from Step Four.
Step Six : That’s it after 5 to 8 minute of process it will reboot. After the reboot, you will see Cydia on your springboard. Now your have a Jailbreaked iPhone on your hand.
Unlock iPhone 3.0.1
1. Once your iPhone is jailbroken, open Cydia.
2. Add repo666.ultrasn0w.com to your sources
3. Install ultrasn0w, and reboot!
4. Now your iPhone should be unlocked.
Tuesday, August 4, 2009
How to listen Tamil radios on your iPhone

1. Connect your iPhone to any local WiFi network.
2. Open Fstream app on your iPhone.
3. Tap on More, under Web click Web Management.
4. Turn on Server Activation.
5. Browse the web address shown in the web management on your PC or Laptop which is connected to same router/network. Enter the user name and password. Default user name and password are admin.
6. Click Manage Your Website.
7. Input the following on each line then click "+" to add your radio stations link.
I have attached here the following radio stations' links.
CMR, CTR, MTR, TTR, GTR, CTBC, IBC, ATBC, 680AM(CANADA)
Note: You can add any radio link right from your iPhone. But I prefer this method to add it quickly and more customs like tamil fonts in the name.
How to view Tamil websites on your iPhone/iPod Touch
Before you proceed for following, please make sure you have installed OpenSSH.
If not, intall it from Cydia. Use WinSCP to connect your iPhone. If you are not sure hwo to SSH to iPhone, visit HERE. Someone has nicely guided.
Install fonts on iPhone
1. Connect to your iPhone using WinSCP via it’s IP address and use username: root, password: alpine
2. Navigate to this folder /System/Library/Fonts/ and overwrite the CGFontCache.plist with the modified CGFontCache.plist file (download here).
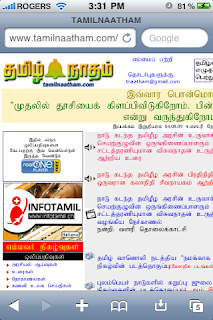
4. Copy all four Tamil fonts file (download here) to the MyFonts folder.
5. Restart/respring to and browse http://www.tamilnaatham.com/ to see the installed fonts work properly.